Using the API#
The Preprocessor
class defines a range of filters that are outlined in the API reference. It’s methods operates on numpy.ndarray
instances, such as the data
of Density
instances. The create_mask
utility on the other hand can be used to define a variety of masks for template matching.
The following outlines the creation of an ellipsoidal mask using create_mask
to create an ellipsoid, in this case a sphere, with radius 15 as mask. create_mask
returns a numpy.ndarray
object, which we use in turn to create a Density
instance. Note that by default, the instances attributes sampling_rate
will be initialized to one unit per voxel, and the origin
attribute to zero. We can write the created mask to disk using Density.to_file
.
from tme import Density
from tme.matching_utils import create_mask
mask = create_mask(
mask_type = "ellipse",
center = (25, 25, 25),
shape = (50, 50, 50),
radius = (15, 15, 15),
sigma_decay = 2
)
Density(mask).to_file("example_gaussian_filter.mrc")
You can visualize the output using your favourite macromolecular viewer. For simplicity, we are showing a maximum intensity projection. The left hand side shows the unfiltered example.mrc
, while the right hand side displays the filtered example_gaussian_filter.mrc
. The degree of smoothing depends on the value of the sigma
parameter.
(Source code
, png
, hires.png
, pdf
)
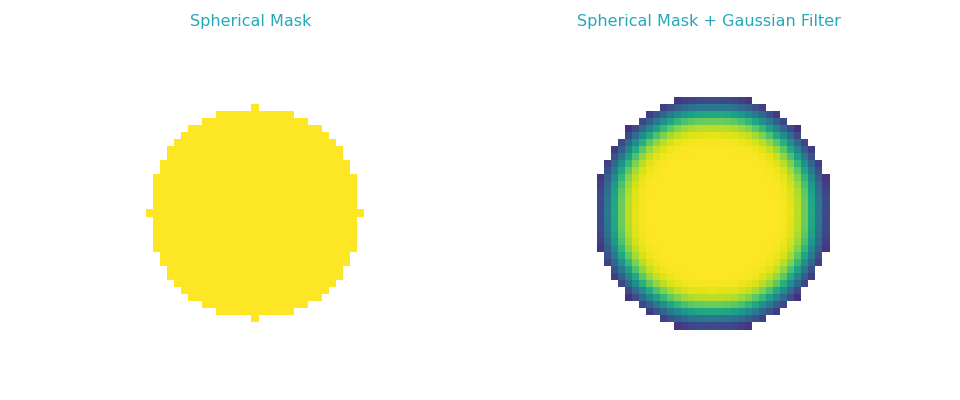
Mask creation and filter application using the API.#
The Preprocessor
, supports a range of other operations such as the creation of wedge masks. The following outlines the creation of a continuous wedge mask assuming an infinite plane using continuous_wedge_mask
.
import numpy as np
from tme import Density, Preprocessor
preprocessor = Preprocessor()
mask = preprocessor.continuous_wedge_mask(
shape = (32,32,32),
opening_axis = 0,
tilt_axis = 2,
start_tilt = 40,
stop_tilt = 40,
# RELION assumes symmetric FFT
omit_negative_frequencies = False
)
Density(mask).to_file("wedge_mask.mrc")
By default, all Fourier operations in pytme assume the DC component to be at the origin of the array. To observe the typical wedge shape you have to shift the DC component of the mask to the array center, for instance by using numpy.fft.fftshift
like so:
import numpy as np
mask_center = np.fft.fftshift(mask)
Density(mask_center).to_file("wedge_mask_centered.mrc")